Raxo
Raxo is a work-in-progress real-time rasterizing software renderer with a highly flexible, full-precision floating-point pipeline. This means that it draws animated objects and scenes using software running on the CPU instead of with hardware on the graphics card.
Raxo is written in templated C++, and I am working on using just-in-time (JIT) compilation of assembly routines to accelerate rendering, by eliminating costly conditionals in inner loops.
GitHub project: http://github.com/GHF/Raxo (git source repository)
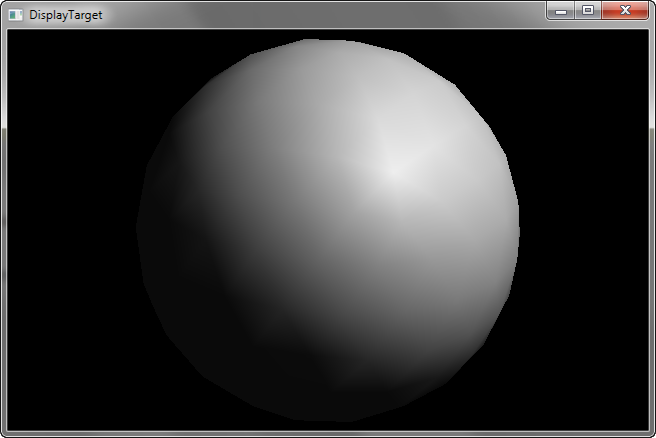
Raxo, currently in heavy development, is a continuation of my work for Stuyvesant’s ML6: Computer Graphics course, taught by Michael Zamansky. While I may later write games or demos with it, it is currently a way for me to fully explore both the “classical” and novel rendering techniques used by and developed by computer graphics researchers and game programmers. I will do so by implementing those techniques myself, in the most badass, impressive, mind-numbingly fast ways I can devise.
Goals §
- Raxo is, above all, a learning tool for myself. It’ll probably become the biggest project I’ve worked on alone. Though this is a rewrite of a bigger group project and I’ve worked on much bigger software projects outside of an academic setting, I have to say that design and architecture is hard.
- This is how it’ll work: I’m going to learn what it is I’m trying to learn (still figuring this out) by deriving and implementing. I’ll gobble up blog posts, research papers, and textbooks, but in the end I have to turn it into code.
- It’s got to be fast. What is computer graphics research if not a never-ending expedient tradeoff between speed and trickery of the eye?
- It’s going to be done right; more right (err, rightly?) than the hardware we have now. I want proper fill rules, subpixel precision where needed, a full floating-point pipeline (that’s right, textures, color buffers, lights—all 128-bit color), the whole works.
- I want to explore techniques published in papers but never implemented in modern hard. I have a flex-function pipeline and no need to release a product, so why not go ahead and play with irregular Z-buffers for shadow mapping or silhouette maps?
Implemented features §
- A sensible C++ architecture and pipeline. Raxo is a library; it’s a collection of loosely-linked classes that form a rendering pipeline, like OpenGL or Direct3D. It takes in geometric data and draws it, but without an animation application or 3D models, it can’t do much
- Coordinate systems with homogeneous coordinates
- 4×4 Matrices and transformations (including a SSE/SSE2 implementation with an amortized cost of about 17 instruction cycles per vector on modern Intel CPUs)
- Full coordinate transformation pipeline as in the OpenGL fixed-function pipeline, from eye space coordinates to viewport coordinates
- Quaternion representation of rotations, including conversions to and from rotation matrices, linear interpolation in spherical space, and linear interpolation in linear space with normalization
- Arbitrary vertex and pixel shaders, with arbitrary number of interpolants (specified at compile time with template parameters)
- OpenGL-style materials/lighting properties
I really hate this sphere now. Its render screw-ups has become a blooper reel for my life.
Work-in-progress §
- A “default” rasterizer that supports most of the features in OpenGL
- Classical Blinn-Phong lighting, with point, directional, and spot lights
- Geometry generators and file loaders (I’ve been staring at the same darn spinning tesselated sphere for more than a year now as a reference model)
- Run-time specified render buffers—Raxo can render to screen using PixelToaster, to file, or to a null target for headless testing
- Converting some inner loops to assembly so they can be generated by AsmJit
- Hand-coded SSE/SSE2/SSE3/SSSE3/SSE4.1 (list would be longer if I had a newer computer) intrinsics to accelerate some key parts
- Camera system
Planned features §
- Perspective correction for interpolants
- Shadow maps and shadow volumes
- External asset loading from files
- Different mesh storage methods
- Precomputed mapped ambient occlusion
- Screen space ambient occlusion
- Tone mapping and gamma correction of output image onto screen
- Depth of field
And beyond… §
- Postprocessing filters (also JIT compiled)
- Image quality (multi-sampling, texture filtering, etc.)
- Things I find in research papers
- ???
- PROFIT! (maybe this will help me find a job?)
Links §
- Chris Hecker’s perspective texture mapping articles – Great for learning proper rasterization rules, subpixel and subtexel precision, linear interpolation of variables, and of course, perspective-correct texturing.
- AsmJit – Terrific runtime assembler. Generates executable functions from assembly, and does higher-level things too, like register allocation/memory spilling for variables. Since the death of SoftWire, this is the best choice for JIT assembly. Google Chromes’s V8 and Safari’s SquirrelFish Extreme JavaScript engines have JIT components as well that can be extracted for use, but of course they are not designed for the same tasks.
- PixelToaster – Cross-platform framebuffer library of champions.
- ompf.org forum – Community for real-time ray tracing enthusiasts. Full of wizardry in assembly, approximations, hardware hacks and of course, floating point color.
- Rasterization on Larrabee – Reading Mike Abrash (author of the Black Book, developer for Quake and Pixomatic) is always inspirational and a bit humbling. Even when he talks about doing things you’ve no desire of ever trying, you can appreciate the effort and ingenuity that goes into it all. Also, Larrabee. Sweet.
- Real Time Rendering – Nice book to have.
- FastFlow – Interesting C++ parallel programming library with different abstraction layers for describing computing problems and solving each in parallel.
- Brown CS123 slides – Slides from course taught by van Dam of Foley and van Dam. The 2009 course supposedly uses an unpublished version of said book.
- SSE Idioms